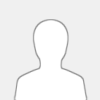 |
Hey, I'm trying to compile mGBA so I can modify it for my specific needs and I'm running into a lot of weird errors. I'm a complete noob when it comes to cmake and MSYS2 and all that and I can't figure out what the problem is.
So I followed the compile instructions on the github readme using MSYS2 until the actual cmake command. For the cmake command I wrote:
Code: cmake -DCMAKE_TOOLCHAIN_FILE=../src/platform/psp2/CMakeToolchain.vitasdk .. -G "MSYS Makefiles"
Cmake printed out a bunch of configuration info but no errors. It recognized the vitasdk binaries properly and everything.
Then, when I enter make, everything seems to go fine with some warnings until 66% at psp2-context.c. At that point, it errors out, spitting out this:
Code: C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c: In function '_setRumble':
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:137:9: error: variable 'state' has initializer but incomplete type
struct SceCtrlActuator state = {
^
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:138:3: warning: excess elements in struct initializer
rumble->current * 31,
^
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:138:3: note: (near initialization for 'state')
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:139:3: warning: excess elements in struct initializer
0
^
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:139:3: note: (near initialization for 'state')
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:137:25: error: storage size of 'state' isn't known
struct SceCtrlActuator state = {
^
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:141:2: warning: implicit declaration of function 'sceCtrlSetActuator' [-Wimplicit-function-declaration]
sceCtrlSetActuator(1, &state);
^
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:137:25: warning: unused variable 'state' [-Wunused-variable]
struct SceCtrlActuator state = {
^
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c: In function 'mPSP2Setup':
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:177:2: warning: implicit declaration of function 'scePowerSetArmClockFrequency' [-Wimplicit-function-declaration]
scePowerSetArmClockFrequency(80);
^
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c: In function 'mPSP2Paused':
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:290:9: error: variable 'state' has initializer but incomplete type
struct SceCtrlActuator state = {
^
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:291:3: warning: excess elements in struct initializer
0,
^
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:291:3: note: (near initialization for 'state')
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:292:3: warning: excess elements in struct initializer
0
^
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:292:3: note: (near initialization for 'state')
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:290:25: error: storage size of 'state' isn't known
struct SceCtrlActuator state = {
^
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:290:25: warning: unused variable 'state' [-Wunused-variable]
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c: In function 'mPSP2Teardown':
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:304:39: warning: unused parameter 'runner' [-Wunused-parameter]
void mPSP2Teardown(struct mGUIRunner* runner) {
^
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c: In function 'mPSP2DrawScreenshot':
C:/msys64/home/Rick/mgba/src/platform/psp2/psp2-context.c:382:16: warning: comparison between signed and unsigned integer expressions [-Wsign-compare]
for (y = 0; y < height; ++y) {
^
make[2]: *** [CMakeFiles/mgba.dir/build.make:1903: CMakeFiles/mgba.dir/src/platform/psp2/psp2-context.c.obj] Error 1
make[1]: *** [CMakeFiles/Makefile2:105: CMakeFiles/mgba.dir/all] Error 2
make: *** [Makefile:150: all] Error 2
For some reason it just really doesn't like SceCtrlActuator
I noticed that psp2-context.c is the first vita-specific file it tries to compile, so I figured it was a problem with vitasdk. I checked the include files to see if SceCtrlActuator was actually defined in the version I had and sure enough, it was defined in ctrl.h which is included properly in psp2-context.c. So...I'm confused as to what's going on. The compiler properly recognized the binaries from the library so how is it not recognizing the headers now? Is my installation of the VitaSDK messed up somehow? I've tried reinstalling the vitasdk and updating it a few times with no change.
Am I just royally screwing up using cmake? Anyone have any ideas? I'd greatly appreciate it! Thanks! Let me know if I need to post any more info to make my problem more clear.
|